Receipt Printing API
This documentation article discusses the Receipt Printing API which is available for the V400m device only
Introduction
The
V400m reader
has a built-in receipt printer which can be triggered by using the receipt
printing API's
requestPrintFromInStoreReader
mutation discussed on this page. The receipt printing API allows for an
API caller to fully customize the text, line items, images, and other
information printed on the V400m built-in receipt printer. The total
contents on a given receipt printing request cannot exceed 122,880 bytes
in size, giving the API caller ample room for a fully customized receipt.
Initiating a printing request
To initiate a printing request using the
requestPrintFromInStoreReader
API mutation you must specify a
readerId
for the V400m device you want to print from and the
contents
that you would like to print on the receipt. For the request to be
successful there must be a minimum of 1 content and a maximum of 500
contents in the request. Each content represents a text line or an image
to be printed on the receipt. The
contents
for any given receipt request cannot exceed 122,880 bytes in size. Within
the
contents
you must specify the
type of input
being either a text or an image.
Text Inputs
When using the
text input
to write a text line on the receipt you may specify the text
value
which is the actual text being printed, the
alignment
of the text, and the
endOfLineFlag
to determine when a given text line should be ended to start a new line
with your next
content
. The default styling will apply to the text unless
you also specify the
textDecoration
which controls the emphasis on the text, the
fontWeight
, the fontStyle
, and the
fontSize
.
Image Inputs
When using the
image input
to print images such as business logos or QR codes the image must be in a
PNG image format and cannot exceed 76,800 bytes in size. The PNG image
must also be base64 encoded before passing the base64 encoded string in
the
value
API field. You may also specify the
endOfLineFlag
to determine when the next text line or image line should begin, as well
as the
alignment
of the image to be printed. For optimization, the ideal image width is 300
pixels.
Example print request
- GraphQL Mutation
- GraphQL Variables
- Sample API Response
mutation RequestPrintFromInStoreReader($input: RequestPrintFromInStoreReaderInput!) {
requestPrintFromInStoreReader(input: $input) {
clientMutationId
id
reader {
id
name
status
location {
id
name
address {
streetAddress
extendedAddress
locality
region
postalCode
countryCode
}
}
}
status
}
}
Once you make the initial request you must also begin a context ID polling process so that your application can determine whether the printing action was successful or not.
Example context ID polling request
We recommend polling every 1-2 seconds to get the final result. There is a default timeout of 30 seconds if the print is unsuccessful. Follow our general node query polling logic documented in our payment processing documentation.
- GraphQL Mutation
- GraphQL Variables
- Sample API Response
query ID($contextId: ID!) {
node(id: $contextId) {
... on RequestPrintInStoreContext {
__typename
id
status
errors {
message
errorCode
}
}
}
}
Printing Receipts in Offline Mode
If you are implementing the offline processing feature then you may also want to be able to print receipts while in offline mode. This is supported, see the example offline receipt printing requests below:
Example offline printing request
- GraphQL Mutation
- GraphQL Variables
- Sample API Response
mutation RequestPrintFromInStoreReader($input: RequestPrintFromInStoreReaderInput!) {
requestPrintFromInStoreReader(input: $input) {
clientMutationId
id
status
}
}
Example offline printing node query
Follow the offline processing node query polling logic documented in our offline processing feature documentation.
- GraphQL Mutation
- GraphQL Variables
- Sample API Response
query ID($contextId: ID!) {
node(id: $contextId) {
... on RequestPrintInStoreContext {
__typename
id
status
errors {
message
errorCode
}
}
}
}
Sample Receipt Template
Since the receipt is entirely customizable we have put together a sample template that you may use as a baseline to begin your custom receipt development. This sample template is meant to be generic but also includes key EMV receipt data (sample EMV compliant receipt reference) which is recommended to be included in your receipt to maintain EMV receipt compliance.
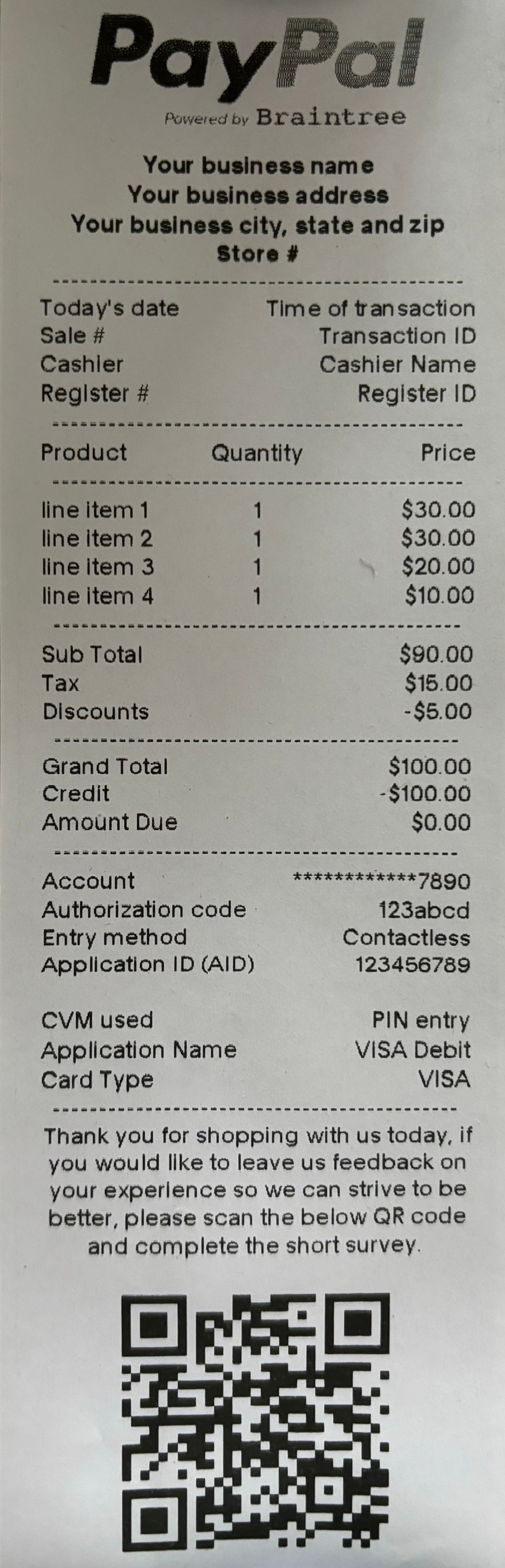
The sample API template can be downloaded as a Postman API collection below:
Important tips for integrating with the Receipt Printing API
- Make sure to handle Receipt Printing API-specific error codes 96733 - 96737
Only PNG image format is supported in the image input, and images must be base64 encoded
The ideal image width is 300 pixels
The hard-coded timeout of an unsuccessful print is 30 seconds
For line spacing use the
text
content input with"value": " "
, you can increase thefontSize
for larger spacingReceipt Printing API is supported in offline mode