Vaulted Payments
Vaulted payments with PayPal account will allow you to charge the account in the future without requiring your customer to be present during the transaction or re-authenticate with PayPal when they are present during the transaction.
The SSL certificates for Braintree Mobile (iOS and Android) SDKs are set to expire on March 30, 2026. This will impact existing versions of the SDK in published versions of your app. To reduce the impact, upgrade the iOS SDK to version 6.17.0+ for the new SSL certifications.
If you do not decommission your app versions that include the older SDK versions or force upgrade your app with the updated certificates by the expiration date, 100% of your customer traffic will fail.
The Vault is used to process payments with our recurring billing feature, and is also used for non-recurring transactions so that your customers don't need to re-enter their information each time they make a purchase from you.
Vaulted payments with PayPal account allows you to charge the account in the future without requiring your customer’s presence during the transaction.
The vaulted payment flow lets the user:
Select or add shipping addresses in the PayPal account
Select or add funding instruments in the PayPal account
Two-factor authentication support (currently only for US, UK, CA, DE, AT, and AU)
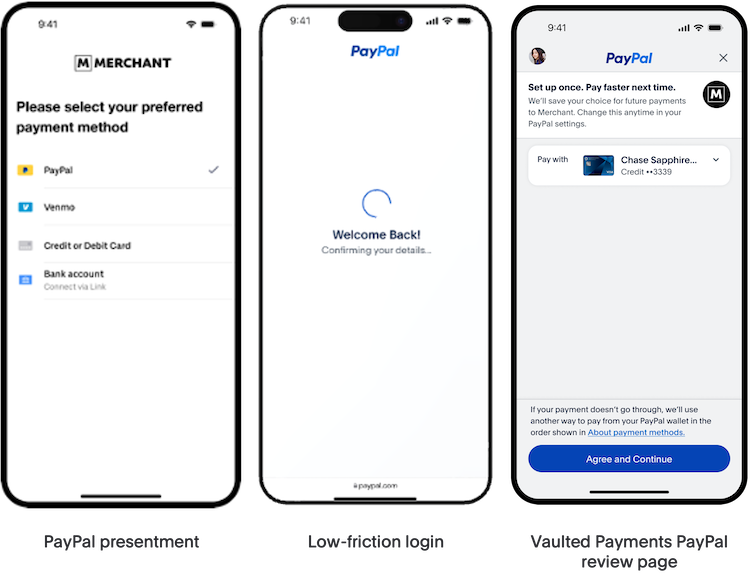
Typical use cases for the vaulted payment flow:
- Faster payments for repeat customers
- Subscriptions
- Recurring billing (e.g. automatic top-up or usage based charges)
Launch the flow
- swift
// Pass the user's email on the vault request
let vaultRequest = BTPayPalVaultRequest()
vaultRequest.userAuthenticationEmail = "\<USER_EMAIL>"
Invoking the Vault flow
- Swift
class MyViewController: UIViewController {
var braintreeClient: BTAPIClient?
func startCheckout() {
// Example: Initialize BTAPIClient, if you haven't already
braintreeClient = BTAPIClient(authorization: "<#CLIENT_AUTHORIZATION#>")!
let payPalClient = BTPayPalClient(apiClient: braintreeClient!)
let request = BTPayPalVaultRequest()
request.billingAgreementDescription = "Your agreement description" // Displayed in customer's PayPal account
payPalClient.tokenize(request) { (tokenizedPayPalAccount, error) -> Void in
if let tokenizedPayPalAccount = tokenizedPayPalAccount {
print("Got a nonce: \(tokenizedPayPalAccount.nonce)")
// Send payment method nonce to your server to create a transaction
} else if let error = error {
// Handle error here...
} else {
// Buyer canceled payment approval
}
}
}
}
Collecting device data
Collecting device data from your customers is required when initiating non-recurring transactions from Vault records. For instructions, see the Premium Fraud Management Tools Guide.
App Switch
The App Switch initiative will enable PayPal users who have a PayPal installed on their phone to complete payments on the PayPal app when it is available.
In this experience the SDK will attempt to switch to the PayPal App after calling tokenize if the PayPal App is installed and the user meets eligibility requirements. If the switch into the PayPal App cannot be completed, we will fall back to the ASWebAuthenticationSession experience.
BTAPIClient needs to be instantiated during the app load phase instead of during on button click.
Get the SDK
The PayPal App Switch is part of the BraintreePayPal module in the Braintree SDK. This module can be pulled into your app via all of the currently supported package managers.
Cocoapods
In your Podfile, add the dependency for the PayPal Mobile Checkout module:
- Ruby
pod 'Braintree/PayPal'
Swift Package Manager
Include the BraintreeCore, BraintreePayPal, and PayPalDataCollector frameworks.
Carthage
Include the BraintreeCore, BraintreePayPal and PayPalDataCollector frameworks.
Allowlist PayPal URL Scheme
You must add the following to the queries schemes allowlist in your app's info.plist:
- XML
<key>LSApplicationQueriesSchemes</key>
<array>
<string>paypal-app-switch-checkout</string>
</array>
Set Up Universal Links
In order to use the PayPal App Switch flow your application must be set up for Universal Links. You will need to use a custom path dedicated to Braintree app switch returns. This URL must also be added to your app association file with wildcards allowed to ensure we receive the expected data on return.
apple-app-site-association
may look like the following:
- JSON
{
"applinks": {
"details": [
{
"appID": "com.your-app-id",
"paths": [
"/braintree-payments/*"
]
}
]
}
}
Register Universal Link in Control Panel
Before using this feature, you must register your Universal Link domain in the Braintree Control Panel:
- Log into your Control Panel (e.g. Sandbox, or Production).
- Click on the gear icon in the top right corner. A drop-down menu will open.
- Click Account Settings from the drop-down menu.
- Scroll to the Payment Methods section.
- Next to PayPal, click the Options link. This will take you to your linked PayPal Account(s) page.
- Click the View Domain Names button. This will take you to the PayPal Domain Names page.
- Note: If you have a single PayPal account, it will be at the bottom of the page. If you have multiple PayPal accounts, it will be at the top right of the page.
- Click the + Add link on the top right of the page or scroll to the Specify Your Domain Names section.
- In the text box enter your list of domain names separated by commas.
- Note: The value you enter must match your fully qualified domain name exactly – including the "www." if applicable.
- Click the Add Domain Names button.
- If the domain registration was successful for all the domain names listed in the text box, a banner will display the text "Successfully added domains". The registered domain names will be displayed in alphabetical order under the + Add button.
- If the registration was not successful for any of the domain names listed in the text box, a banner will display a list of domain names that failed qualified domain name validation along with their reasons for rejection. Any domain names that were successfully registered will be displayed in alphabetical order under the + Add button.
- Note: You can re-enter the rejected domain names in the text area with the corrections applied.
Set Universal Link in SDK
You will need to use the following BTPayPalClientinit to set your Universal Link:
- Swift
let apiClient = BTAPIClient(authorization: <#CLIENT_AUTHORIZATION#>)
let payPalClient = BTPayPalClient(
apiClient: apiClient, // required
universalLink: URL(string: "https://my-universal-link.com/braintree-payments")! // required
)
Handle App Context Switching
Then pass the URL to Braintree for completion of the flow.
If you’re using SwiftUI, you must add the onOpenURL(perform:) modifier to your ContentView, and then call BTAppContextSwitcher's handleOpen(_:) method.
- Swift
.onOpenURL { url in
BTAppContextSwitcher.sharedInstance.handleOpen(url)
}
If you're using UISceneDelegate
(introduced in iOS 13), call the handleOpen(_\:)
method from within the scene(_:continue:)
scene delegate method.
- Swift
func scene(_ scene: UIScene, continue userActivity: NSUserActivity) {
if let returnURL = userActivity.webpageURL, returnURL.path.contains("/my-dedicated-braintree-path") {
BTAppContextSwitcher.sharedInstance.handleOpen(returnURL)
}
}
Otherwise, if you aren't using UISceneDelegate, call the handleOpen(_:)
method method from within the application(_:continue:restorationHandler:) app delegate method.
- Swift
func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool {
if let returnURL = userActivity.webpageURL, returnURL.path.contains("/my-dedicated-braintree-path") {
BTAppContextSwitcher.sharedInstance.handleOpen(returnURL)
}
}
Invoking the PayPal App Switch flow
Opt in to the App Switch flow
Construct a BTPayPalVaultRequest or a BTPayPalCheckoutRequest with enablePayPalAppSwitch set to true and a userAuthenticationEmail included. Use this with your BTPayPalClient from above to call BTPayPalClient.tokenize(_:completion:) to launch the PayPal App Switch flow.
An example integration might look like this:
- Swift
import UIKit
import BraintreePayPal
class MyViewController: UIViewController {
var apiClient: BTAPIClient!
var payPalClient: BTPayPalClient!
override func viewDidLoad() {
super.viewDidLoad()
apiClient = BTAPIClient(authorization: <#CLIENT_AUTHORIZATION#>)
payPalClient = BTPayPalClient(
apiClient: apiClient,
universalLink: universalLink: URL(string: "https://my-universal-link.com/braintree-payments")! // required for this flow
)
}
private func payPalButtonTapped() {
// vault flows
let request = BTPayPalVaultRequest(
userAuthenticationEmail: "sally@gmail.com",
enablePayPalAppSwitch: true
)
// one time checkout flows
let request = BTPayPalCheckoutRequest(
amount: amount
userAuthenticationEmail: "sally@gmail.com",
enablePayPalAppSwitch: true
)
// userAuthenticationEmail and enablePayPalAppSwitch are required for this flow
// Configure other values on 'request' as needed.
payPalClient.tokenize(request) { payPalNonce, error in
if let payPalNonce {
// send payPalNonce.nonce to server
} else {
// handle error
}
}
}
}
Shipping address
Shipping addresses may or may not be collected during the PayPal Vault flow. However, if you choose to collect shipping addresses yourself, it can be passed along with the server side Transaction.Sale call. Look at the Server-side page for more information.
Country and language support
PayPal is available to merchants in all countries that we support and to customers in 140+ countries.
Currency presentment
In the Vault flow itself, the transaction currency and amount are not displayed to the customer. It is up to you to display these details in your checkout flow somewhere (e.g. cart page, order review page, etc.). Our Server-Side guide outlines which currencies are supported for PayPal transactions.