Advanced Options
Add *.paypal.com to your Content Security Policy (skip if using Hosted Card Fields)Configure your Content Security Policy
Content Security Policy is a feature of web browsers that mitigates cross-site scripting and other
attacks. By limiting the origins of resources that may be loaded on your page, you can maintain
tighter control over any potentially malicious code. While browser support is relatively limited, we
recommend considering the implementation of a CSP when available. Include the following directive in
your policy:
connect-src: https://*.paypal.com https://*.paypalobjects.com https://*.braintreegateway.com https://*.braintree-api.com
font-src: https://*.paypalobjects.com
frame-src: https://*.paypal.com https://*.braintreegateway.com
img-src: https://*.paypal.com https://*.paypalobjects.com
script-src: https://*.paypal.com https://*.paypalobjects.com https://*.braintreegateway.com
style-src: unsafe-inline
Specify locale
To specify the locale in which the Fastlane components should be rendered, you can set the locale
after initializing the fastlane component.fastlane.setLocale("en_us"); // en_us is the default value
Fastlane supports the following languages: en_us
(default)es_us
fr_us
zh_us
Rendering Fastlane Watermark**
There are two ways to recommend Fastlane Watermark :
Watermark without the info icon
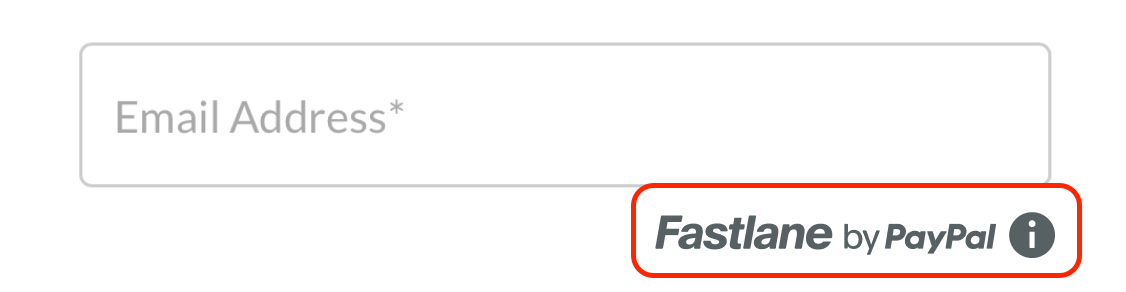
Watermark with the info icon
- HTML
<!-- add a div where the watermark will be rendered -->
<div id="watermark-container">
<img src="https://www.paypalobjects.com/fastlane-v1/assets/fastlane-with-tooltip_en_sm_light.0808.svg" />
</div>
- JavaScript
const fastlaneWatermark = (await fastlane.FastlaneWatermarkComponent({
includeAdditionalInfo: true
}));
await fastlaneWatermark.render("#watermark-container");
Watermark without the info icon
You can use the following code to render the watermark without the "info" icon: HTML Sample
- HTML
<!-- add a div where the watermark will be rendered -->
<div id="watermark-container">
<img src="https://www.paypalobjects.com/fastlane-v1/assets/fastlane_en_sm_light.0296.svg" />
</div>
- JavaScript
const fastlaneWatermark = (await fastlane.FastlaneWatermarkComponent({
includeAdditionalInfo: false
}));
await fastlaneWatermark.render("#watermark-container");
Optimization: Preload watermark assets
For a better payer experience of Fastlane, it is recommended to preload the watermark asset by
adding the following code to the head
section of the page. Even though this is
optional, we highly recommend it.
- HTML
<link rel="preload" href="https://www.paypalobjects.com/fastlane-v1/assets/fastlane-with-tooltip_en_sm_light.0808.svg" as="image" type="image/avif" />
<link rel="preload" href="https://www.paypalobjects.com/fastlane-v1/assets/fastlane_en_sm_light.0296.svg" as="image" type="image/avif" />
Shipping Address Guidelines
Only supports US addresses:- While Fastlane is only available with billing addresses in the US, the shipping address can be any location that your site supports shipping to.
- For information on how to limit the available shipping addresses, please see the reference types section.
- If the payer adds a new address, ensure you can send that address in the server-side transaction.sale() request.
- HTML
<!-- Div container for the Payment Component -->
<div id="card-container"> </div>
<div id="selected-card"> <!-- render selected card here --> </div>
<a href="" id="change-card-link"> Change card </a>
<div id="watermark-container">
<img src="https://www.paypalobjects.com/fastlane-v1/assets/fastlane_en_sm_light.0296.svg" />
</div>
<!-- Submit Button -->
<button id="submit-button"> Submit Order </button>
- javascript
const name = profileData.name;
const shippingAddress = profileData.shippingAddress;
const card = profileData.card;
var selectedCardForCheckout = card;
if (memberAuthenticatedSuccessfully && card) {
// refer to Lookup & Authenticate section for details
// render the card here
// render Fastlane watermark
// render a change button and call profile.showCardSelector() when it is clicked
} else {
// User is a guest, failed to authenticate or does not have a card in the profile.
// render the card fields
const fastlaneCardComponentOptions = {
fields: {
phoneNumber: {
// Example of how to prefill the phone number field in the FastlaneCardComponent
prefill: "4026607986"
},
cardholderName: {
// Example of disabling and prefilling the cardholder name field
prefill: "John Doe",
enabled: false
}
},
styles: {
root: {
// specify styles here
backgroundColorPrimary: "#ffffff"
}
}
};
const fastlaneCardComponent = await fastlane.FastlaneCardComponent(fastlaneCardComponentOptions);
fastlaneCardComponent.render("#card-container");
}
// Handle changes to the card selection
const changeCardButton = document.getElementById("change-card-button");
changeCardButton.addEventListener("click", async () => {
const { selectionChanged, selectedCard } = await profile.showCardSelector();
if (selectionChanged) {
// selectedCard contains the new card
// selectedCard.id contains the paymentToken
// selectedCard.paymentSource.card contains more details such as last 4
selectedCardForCheckout = selectedCard;
// re-render the selected card UI if required
} else {
// selection modal was dismissed without selection
}
});
// Handle form submission
const submitButton = document.getElementById("submit-button");
submitButton.addEventListener("click", async () => {
var paymentToken = null;
// if the Card Component is rendered,
// pass the billing address and get the paymentToken
if (selectedCardForCheckout) {
paymentToken = selectedCardForCheckout.id;
} else {
paymentToken = await fastlaneCardComponent.getPaymentToken({
billingAddress: {
cardholderName: "John Doe",
streetAddress: "2211 North 1st St",
locality: "San Jose",
region: "CA",
postalCode: "95131",
// you can also use the countryCodeAlpha3 or countryCodeNumeric formats
countryCodeAlpha2: "US"
}
});
}
// Send the paymentToken and previously captured device data to server
// to complete checkout
});
Field name | Description | Link |
---|---|---|
device_data | An identifier that helps prevent fraud and ensures the highest authorization rates. | Link to the documentation |
billing | The billing object contains fields related to the payer’s billing information. | Link to the documentation |
customer.firstName | The payer’s first name. | Link to the documentation |
customer.lastName | The payer's last name. | Link to the documentation |
customer.email | The payer’s email address. | Link to the documentation |
Store pick-up Integration
If the buyer is picking up an item from a store-front, then the shipping type should be modified
accordingly. Ensure
shipping method
is set to pickupInStore or
shipToStore to ensure that the buyer profiles don’t get created
with the address of your store as their shipping address.
Vaulting
- Transact and Vault:
- Vault and transact:
PayPal Members without a Fastlane Profile
PayPal members without a Fastlane profile do not require any additional handling within your
integration. Our client SDK handles this use case for you in the following ways:- After performing the lookupCustomerByEmail method, we will return a customerContextId as if this were a Fastlane member.
- Trigger the triggerAuthenticationFlow method, and our SDK will display a call to action to the buyer explaining that he/she can create a Fastlane profile populated with information from his/her PayPal account with one click.
- If the consumer clicks yes, we will return profileData exactly as we would for a Fastlane member.
- If the consumer closes the dialog, we will return an empty profileData object and you will handle this as you would any Fastlane guest.