Drop-in UI
Setup and Integration
Configuration
To use the Drop-in UI, you'll need to get a tokenization key from the Control Panel or you can generate a client token on your server.
Upgrade to JavaScript v2.17 or higher to use a tokenization key, a static form of client authorization obtained from the Control Panel.
Client-side implementation
Configure the container and form where the Drop-in UI will add the payment method nonce. Make sure to replace CLIENT-AUTHORIZATION with your generated client token.
- html
<form>
<div id="dropin-container"></div>
</form>
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container'
});
braintree.setup requires Braintree.js, which adds a hidden input named payment_method_nonce to your form. As a result, it is required for container to be inside a form. When your user submits the form, you can use the nonce to create transactions or customers, or to store existing customers in the Vault. To learn more about client configuration, visit our setup guide.
PayPal
If you have PayPal configured, you will see a PayPal button with no additional configuration. By default, you will be setup to use our Vault flow. If you would like to use Checkout with PayPal you must pass in singleUse, amount and currency:
- html
<form>
<div id="dropin-container"></div>
</form>
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container',
paypal: {
singleUse: true,
amount: 10.00,
currency: 'USD'
}
});
Fields
The Drop-in UI provides several fields.
- Credit Card
- Expiration Date
- CVV
- Postal Code
CVV and Postal Code are rendered conditionally according to your AVS and CVV Settings.
Options
Container
At a minimum, a container must be provided in order to display the inline form. This container may be an ID, DOM node, or jQuery object (such as $("#container")). Braintree.js will attach its behavior to the closest parent form. The container must be a child of a form.
- html
<form>
<div id="dropin-container"></div>
</form>
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container'
});
Form
If you want Braintree.js to attach its behavior to a form other than the closest parent form (default), you may specify the id of the target form in the options:
- html
<div id="dropin-container"></div>
<form id="checkout-form">
<input type='submit' value='Pay'/>
</form>
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container',
form: 'checkout-form'
});
PayPal Checkout button
If you're not a fan of the PayPal button that comes standard with Drop-in, there's another option: in JS versions 2.18.0+, you can use the PayPal Checkout button instead:
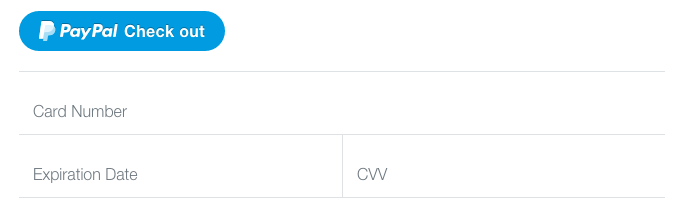
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container',
paypal: {
button: {
type: 'checkout'
}
}
});
Callbacks
The callback options available in the Drop-in integration apply to all Braintree.js integrations. However, the payloads returned may differ slightly depending on the use case. For more information about these top-level callbacks, see the JavaScript SDK reference.
onPaymentMethodReceived
This is called when a payment_method_nonce has been generated by Drop-in (as the result of a form submission). It will be called with a paymentMethod object, which contains the nonce as a string. For more information on this callback and its payload, see Setup method options.
If onPaymentMethodReceived is defined, Braintree.js will not add a hidden input to your form, inject the nonce into that hidden input, or submit the form. Upon receiving a nonce, it is up to you to send data to your server as you see fit.
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container',
onPaymentMethodReceived: function (obj) {
// Do some logic in here.
// When you're ready to submit the form:
myForm.submit();
}
});
onReady
onReady fires when the Braintree Drop-in has fully loaded and is ready to be interacted with by the user. Properties are available in the setup method options reference.
onError
As with onPaymentMethodReceived, the general usage of the onError callback is documented in the setup method options reference.
Validation errors
When client-side validation fails in Drop-in, onError will fire with a VALIDATION type. This error payload will also present information about which fields were invalid as well as whether or not they were empty.
Key | Type | Description | ||||||
type | String | VALIDATION | ||||||
message | String | 'Some payment method input fields are invalid.' | ||||||
details | Object |
An object containing specific details of the error.
|
Key | Type | Description |
fieldKey | String |
A string representing the field which failed validation. It will match one of the following:
- number - cvv - expiration - postalCode |
isEmpty | Boolean | This will be true if the associated input is empty. |
Server-related errors
When a server-related error occurs, onError will fire with a SERVER type. Scenarios in which this may occur:
- The customer's browser can't connect to Braintree.
- The card fails verification and can't be saved to a customer's Vault record. This will only happen if the client token was generated with a
customer_id
and card verification is enabled (either in the Control Panel or with theverify_card
option).
Server-related errors are provided to onError
in version 2.23.0+ of our JavaScript SDK.
Key | Type | Description |
type | String | SERVER |
message | String | An error message returned by the server. |
details | Object | An object containing specific details of the error. This will be passed directly from Braintree's gateway and varies depending on the error. |
- JavaScript
braintree.setup('CLIENT-AUTHORIZATION', 'dropin', {
container: 'dropin-container',
onError: function (obj) {
if (obj.type == 'VALIDATION') {
// Validation errors contain an array of error field objects:
obj.details.invalidFields;
} else if (obj.type == 'SERVER') {
// If the customer's browser can't connect to Braintree:
obj.message; // "Connection error"
// If the credit card failed verification:
obj.message; // "Credit card is invalid"
obj.details; // Object with error-specific information
}
}
});
Next steps
- Read the Set Up Your Server guide to learn about our server SDKs and how to send a nonce to your server
- Explore the ways to customize the appearance and functionality of Drop-in
- Learn how to manage different payment methods