Transactions
- Get authorization to collect money from a customer by creating a sale.
- Collect the money from an authorized sale by submitting the transaction for settlement.
- Undo a transaction before it settles by creating a void.
- Return previously collected money to a customer by creating a refund.
- Search for or find transactions you've created.
- Take more advanced actions depending on your setup.
Status
The transaction
status
indicates the current stage in the transaction lifecycle. Refer to the following diagram, or
see all possible statuses with their explanations
in the reference.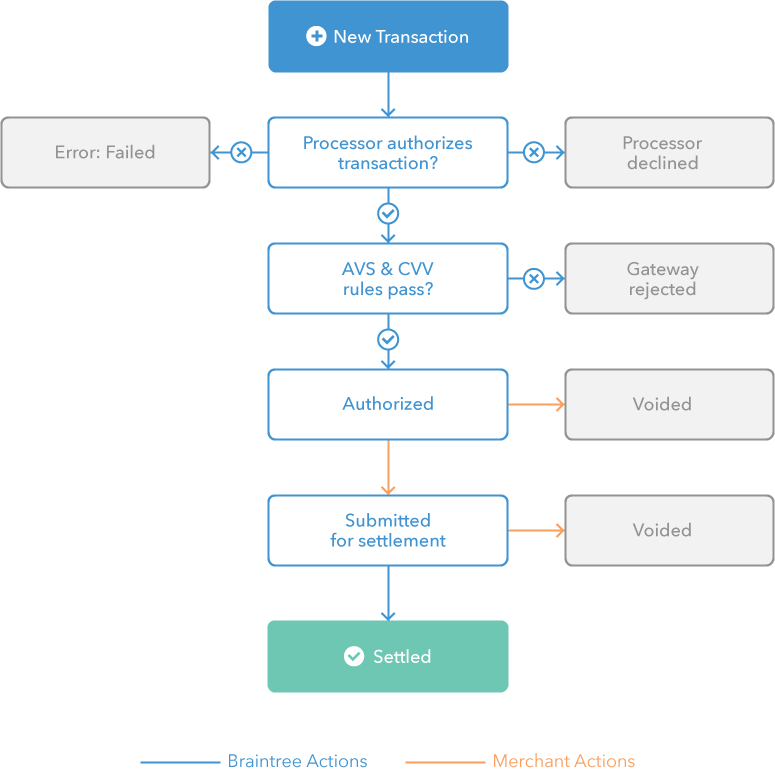
Settlement
To collect money, you'll need to submit the sale transaction for settlement. You can do this in two
ways:- When creating a transaction, use the options.submitForSettlement option:
- Callbacks
- Promises
gateway.transaction.sale({
amount: "10.00",
paymentMethodNonce: nonceFromTheClient,
deviceData: deviceDataFromTheClient,
options: {
submitForSettlement: true
}
}, (err, result) => {
if (result.success) {
// See result.transaction for details
} else {
// Handle errors
}
});
- Callbacks
- Promises
gateway.transaction.submitForSettlement("theTransactionId", (err, result) => {
if (result.success) {
const settledTransaction = result.transaction;
} else {
console.log(result.errors);
}
});
notFoundError
. The transaction must be authorized to submit for settlement. Learn more about submitting
authorized transactions for settlement in our
Managing Authorizations
support article.
Validations
If you provide invalid or malformed transaction details, the gateway returns
validation errors on the transaction. You may also receive other types of validation errors on any additional information associated
with the transaction, including the payment method, customer, and address.
Note
For certain account setups, we recommend that merchants collect and pass billing address
information when storing payment methods or creating transactions. Passing billing address details
(at least the postal code) can help increase the likelihood of a successful authorization. To
learn more about your specific account setup,
contact us.
Disputes
Depending on your account setup, when a customer files a chargeback or other dispute with their bank
or card network, you can retrieve those details from the
transaction response object. Each
transaction response object has a
disputes
property that is an array of zero or more disputes. You can also
search for disputed transactions by the dispute date
. After you've located a disputed transaction, how you handle it depends on your banking partner.
Learn more about chargebacks and retrievals in our support articles.Learn more
See more documentation on transactions:- Set up a basic PayPal Braintree integration to create a sale transaction using our Get Started guide.
- Processor responses on authorization and settlement requests.
- Gateway rejections and how to check the reason for a rejection.
- Supporting sales in multiple currencies by specifying a merchant account ID.
- Differences between refund, void, and detached credit transactions.
- Transactions with 3D Secure.
- Sandbox testing details including test values for creating sandbox transactions.