Onboard sellers before payment
Last updated: Apr 2nd, 11:40pm
How it works
You can connect your sellers with PayPal before they accept PayPal payments from payers on your platform.
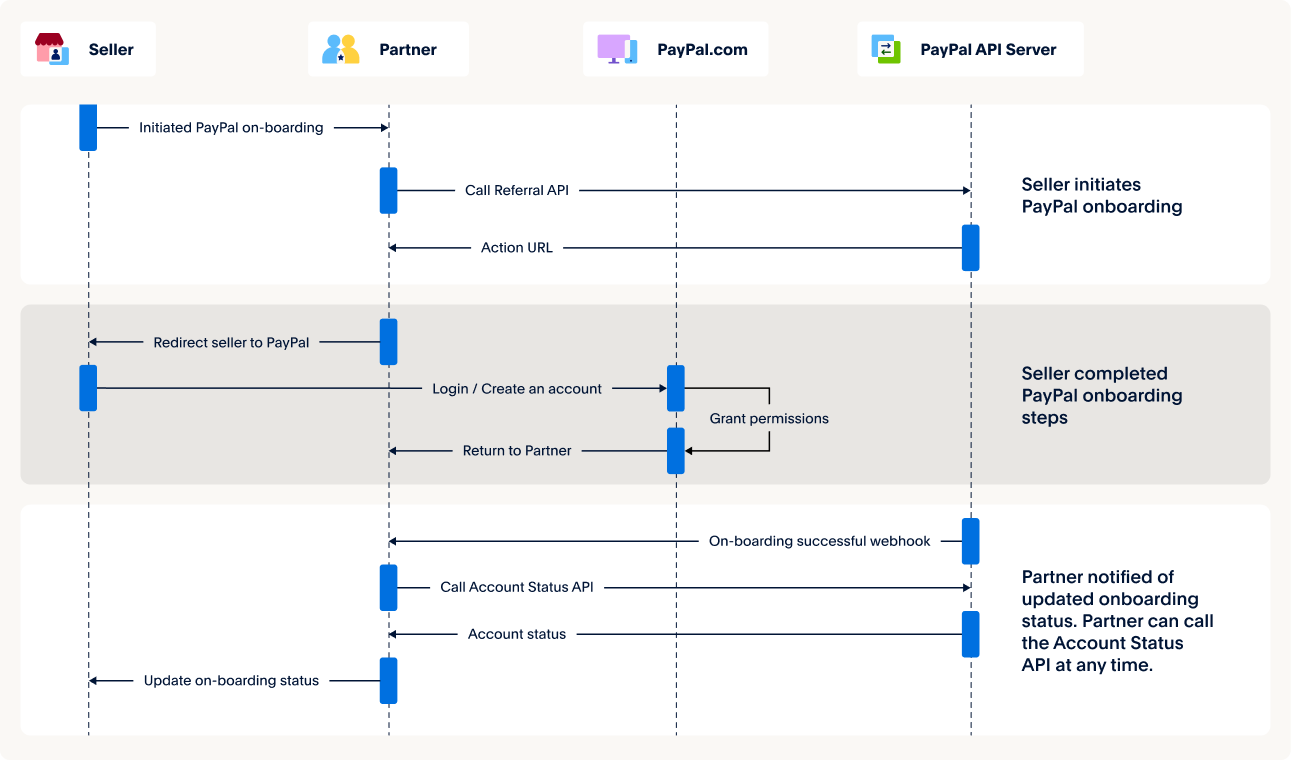
Know before you code
RequiredTo use this integration, you must:
- You must be an approved partner to use this integration.
- You must have an access token.
- This integration uses the Partner Referrals API
- Inform your sellers of PayPal's Seller Protection policy, so they are aware of use cases that invalidate that protection, such as shipping to an address other than the one in the transaction confirmation.
Set up webhooks
Webhooks are HTTP callbacks that receive notification messages for events. You can subscribe to webhooks from the developer portal or by making an API call.
You’ll need to subscribe to the following webhooks for onboarding:
MERCHANT.ONBOARDING.COMPLETED
: This webhook is triggered when the seller has fulfilled all onboarding required and has given you permissions.MERCHANT.PARTNER-CONSENT.REVOKED
: This webhook is triggered when the seller has removed your permissions.
To subscribe to webhooks from your developer portal:
- Select Apps & Credentials.
- Select the name of your REST app.
- Go to Sandbox Webhooks > Add Webhook.
- Enter the URL for your listener.
- Add the event name by clicking the associated check boxes and select Save.
To subscribe to webhooks by making an API call, see Webhooks for the sample requests and responses.
Generate a signup link
Pass your seller’s information in the Partner Referrals API call. The PayPal signup flow will automatically fill with your seller’s information. Generate a signup link that redirects your sellers to sign up with PayPal.
Modify the code
Copy the sample request and modify it as follows:
- Replace
ACCESS-TOKEN
with your access token. - Set
products
to the type of payment you want for your sellers. The products array value determines which payment types your sellers can accept. For more information, seeproducts
in the Partner Referrals API page.The 2 payment types include:
EXPRESS_CHECKOUT
includes debit and credit cardsPPCP
includes the PayPal button and advanced credit and debit cards. Sellers can also accept alternative payment methods such as Pay upon Invoice.
Optional: Pass a tracking ID in the
TRACKING-ID
field. You can use this ID to track the status of your seller as they complete onboarding. For more information, seeTRACKING-ID
in the List seller tracking information page.
Sample request and response
- Sample request
- Sample response
1curl -v -X POST https://api-m.sandbox.paypal.com/v2/customer/partner-referrals \2 -H "Content-Type: application/json" \3 -H "Authorization: Bearer ACCESS-TOKEN" \4 -d '{5"tracking_id": "TRACKING-ID",6"operations": [{7 "operation": "API_INTEGRATION",8 "api_integration_preference": {9 "rest_api_integration": {10 "integration_method": "PAYPAL",11 "integration_type": "THIRD_PARTY",12 "third_party_details": {13 "features": [14 "PAYMENT",15 "REFUND"16 ]17 }18 }19 }20}],21"products": [22 "EXPRESS_CHECKOUT"23],24"legal_consents": [{25 "type": "SHARE_DATA_CONSENT",26 "granted": true27}]28}29'
Step result
A successful request results in the following:
- A return status code of HTTP 201 Created.
- A HATEOAS self link. Make a GET request to this link to retrieve the referral data and to reinitialize the action_url.
- A HATEOAS action_url link. Make a note of this link. The link can be placed in a button or link tag to redirect your sellers to sign up with PayPal. The action_url expires after one use. Refresh the link by making a GET request to the self link or by making another Partner Referrals API call.
Note: If you call GET /v2/customer/partner-referrals/{partner_referral_id}, the response only returns the user data you have passed for the seller. It won’t return any data the seller might have provided on paypal.com.
Add signup link to your site
Insert the action_url
in a button or a link to redirect the seller to the PayPal signup page.
You can also render the PayPal signup flow in a mini-browser by using the following code:
1<div dir="ltr" style="text-align: left;" trbidi="on">2 <script>3 (function(d, s, id) {4 var js, ref = d.getElementsByTagName(s)[0];5 if (!d.getElementById(id)) {6 js = d.createElement(s);7 js.id = id;8 js.async = true;9 js.src = "https://www.paypal.com/webapps/merchantboarding/js/lib/lightbox/partner.js";10 ref.parentNode.insertBefore(js, ref);11 }12 }(document, "script", "paypal-js"));13 </script>14 <a data-paypal-button="true" href="<Action-URL>&displayMode=minibrowser" target="PPFrame">Sign up for PayPal</a>15</div>
Redirect seller to a return URL
After your seller signs up, they are redirected to the return URL you specified in the partner_config_override/return_url
field of the Partner Referrals API. If you don’t specify a return URL, then the seller is redirected to the PayPal dashboard for their account.
The following code sample redirects the seller back to your site in the Partner Referrals API.
Sample request
1https://<Return-URL>?merchantId=<Tracking-ID>&merchantIdInPayPal=<Merchant-ID-In-PayPal>&permissionsGranted=true&accountStatus=BUSINESS_ACCOUNT&consentStatus=true&productIntentId=addipmt&isEmailConfirmed=true&returnMessage=To%20start%20accepting%20payments,%20please%20log%20in%20to%20PayPal%20and%20finish%20signing%20up.
Parameter | Description |
---|---|
merchantId |
The unique ID of the seller in your system that you specified in the Partner Referrals API call in the tracking_id field. |
merchantIdInPayPal |
The merchant ID of your seller's PayPal account. |
permissionsGranted |
A Boolean indicating whether the seller granted you the permissions you specified in the Partner Referrals API call. |
accountStatus |
PayPal sends BUSINESS_ACCOUNT if a business account was created. Otherwise, nothing is sent. |
consentStatus |
A Boolean indicating whether the seller consented to share their credentials with you. |
productIntentId |
Set to addipmt . |
isEmailConfirmed |
A Boolean indicating whether the seller has confirmed their email with PayPal. |
returnMessage |
A hardcoded message containing next steps for the seller to take with PayPal. PayPal only sends this message for business accounts where the email address is not confirmed. PayPal does not send this message for any account where product name is PPCP . |
riskStatus |
The product provisioning status. PayPal only sends this for accounts where product name is PPCP . Possible values are SUBSCRIBED , SUBSCRIBED_WITH_LIMIT , DECLINED , MANUAL_REVIEW , and NEED_MORE_DATA. |
Track seller onboarding status
Onboarding is complete when the seller has done the following:
- Created a PayPal account.
- Granted you permission for the features you set.
- Confirmed the email address of the account.
Make a show seller status call to track your seller’s onboarding status.
You can receive a notification when your seller completes onboarding by subscribing to the MERCHANT.ONBOARDING.COMPLETED
webhook. This webhook is triggered when the seller has fulfilled all onboarding requirements.
Modify the code
Copy the sample request and modify it as follows:
- Replace
ACCESS-TOKEN
with your access token. - Replace
PARTNER-MERCHANT-ID
with your merchant ID.- To get your merchant ID, log in to your PayPal account at paypal.com. Hover over your name or profile icon on the top right and select Account Settings > Business information > PayPal Merchant ID.
- To find the merchant ID of your sandbox account, follow the same instructions on sandbox.paypal.com.
- Replace
SELLER-MERCHANT-ID
with the merchant ID of the seller’s PayPal account.- To get your seller’s merchant ID, look at the
merchantIdInPayPal
query parameter attached to the return URL when the seller is redirected back to your site. You can also query it directly by the tracking ID you specified in the Partner Referrals call by callingGET /v1/customer/partners/partner_id/merchant-integrations?tracking_id={tracking_id}
. For more information, see List seller tracking information.
- To get your seller’s merchant ID, look at the
Sample request and response
In the response, products
is set to PPCP_CUSTOM
.
1curl -v -X GET https://api-m.sandbox.paypal.com/v1/customer/partners/{partner_merchant_id}/merchant-integrations/{seller_merchant_id} \2 -H "Content-Type: application/json" \3 -H "Authorization: Bearer ACCESS-TOKEN"
Step result
A successful request results in the following:
- A return status code of HTTP
200 OK
. - A JSON response body that shows referral data. This response body does not return user data but returns information on whether the merchant is onboarded and is eligible to process transactions.
- The
payments_receivable
is true. - The
primary_email_confirmed
is true. - The
oauth_third_party
contains permissions granted.
Sample Response
In this response, products
is set to Express_Checkout
.
1{2 "merchant_id": "5VT3RDX64P5Z6",3 "tracking_id": "{{tracking_id}}",4 "products": [{5 "name": "EXPRESS_CHECKOUT",6 "status": "ACTIVE"7 },8 {9 "name": "PPCP_STANDARD",10 "vetting_status": "SUBSCRIBED",11 "capabilities": [12 "INSTALLMENTS",13 "SUBSCRIPTIONS",14 "ACCEPT_DONATIONS",15 "PAYPAL_CHECKOUT",16 "GUEST_CHECKOUT",17 "PAYPAL_CHECKOUT_ALTERNATIVE_PAYMENT_METHODS",18 "SEND_INVOICE",19 "QR_CODE",20 "WITHDRAW_FUNDS_TO_DOMESTIC_BANK",21 "PAYPAL_CHECKOUT_PAY_WITH_PAYPAL_CREDIT"22 ]23 }24 ],25 "capabilities": [{26 "name": "INSTALLMENTS",27 "status": "ACTIVE"28 },29 {30 "name": "PAYPAL_CHECKOUT",31 "status": "ACTIVE"32 },33 {34 "name": "SEND_INVOICE",35 "status": "ACTIVE"36 },37 {38 "name": "ACCEPT_DONATIONS",39 "status": "ACTIVE"40 },41 {42 "name": "SUBSCRIPTIONS",43 "status": "ACTIVE"44 },45 {46 "name": "PAYPAL_CHECKOUT_PAY_WITH_PAYPAL_CREDIT",47 "status": "ACTIVE"48 },49 {50 "name": "GUEST_CHECKOUT",51 "status": "ACTIVE"52 },53 {54 "name": "QR_CODE",55 "status": "ACTIVE"56 },57 {58 "name": "PAYPAL_CHECKOUT_ALTERNATIVE_PAYMENT_METHODS",59 "status": "ACTIVE"60 }61 ],62 "payments_receivable": true,63 "legal_name": "Firstname Lastname",64 "primary_email": "test@example.com",65 "primary_email_confirmed": false,66 "oauth_integrations": [{67 "integration_type": "OAUTH_THIRD_PARTY",68 "integration_method": "PAYPAL",69 "oauth_third_party": [{70 "partner_client_id": "B_AhEDQSJmQb9fK4lIyGOQZEwPVBrrk44Fz2vjfXmv2Tpuc1pt16Mb9h6Ua9pnq1SYvjSy1fOuKXFA2NLw",71 "merchant_client_id": "B_AGDGPKGC1P28B65QYRvOmmNe-7JKnEMqIt_H_CMFsXAR_ZuaSLaQBtIKiatmJsv5_ZtxLvp_qPkptoHg",72 "scopes": [73 "https://uri.paypal.com/services/payments/realtimepayment",74 "https://uri.paypal.com/services/reporting/search/read",75 "https://uri.paypal.com/services/payments/refund",76 "https://uri.paypal.com/services/customer/merchant-integrations/read",77 "https://uri.paypal.com/services/payments/payment/authcapture"78 ]79 }]80 }],81 "primary_currency": "USD",82 "country": "US"83}
Sample Response
In this response, products
is set to PPCP_CUSTOM
.
1{2 "merchant_id": "CG5RZJV4NR5P4",3 "tracking_id": "1537989077589",4 "products": [5 {6 "name": "PPCP_CUSTOM"7 }8 ],9 "payments_receivable": true,10 "primary_email_confirmed": true,11 "products.vetting status": "SUBSCRIBED",12 "capabilities[name==CUSTOM_CARD_PROCESSING].status": "ACTIVE",13 "capabilities[name==CUSTOM_CARD_PROCESSING].limits": "undefined",14 "oauth_integrations": [{15 "integration_type": "OAUTH_THIRD_PARTY",16 "integration_method": "PAYPAL",17 "oauth_third_party": [18 {19 "partner_client_id": "Af1bGDNgFBtbJvzEkG25zt4SoNQQ3ustiLm84GWXxe8nq_HE_0wCQ9SH8M1ScmSBURBIzPiCjr5gu-Dq",20 "merchant_client_id": "AQ7u3fJkUH4cdCEBidsh5U_F1RQCGjglJQdNEEsXCzvQsGsS5MmC8Dk7_ug_IlkUASJezaGqQxQQfVhQ",21 "scopes": ["https://uri.paypal.com/services/payments/realtimepayment", "https://uri.paypal.com/services/payments/refund", "https://uri.paypal.com/services/payments/payment/authcapture"]22 }23 ]24 }]25}
URL onboarding
Use URL onboarding only if you have already onboarded with URL onboarding or if you have a specific use case for URL onboarding. With URL onboarding, sellers are required to complete all signup fields. Sellers must also have a PayPal business account. Casual sellers are not supported.
Note: For new integrations, use the Partner Referrals API to onboard your sellers.
Example URL
1https://www.paypal.com/bizsignup/partner/entry?&partnerClientId=<var><partnerClientId></var>&partnerId=<var><merchantId></var>&partnerLogoUrl=<var>&<partnerLogoUrl></var>&returnToPartnerUrl=<var><returnToPartnerUrl></var>&product=ppcp&integrationType=TO&features=PAYMENT,REFUND</var>
Reference
Parameter | Description |
---|---|
channelId |
Set to partner . |
partnerId |
The merchant ID of your PayPal account. To find the merchant ID of your PayPal account, log in to your PayPal account at paypal.com. Hover over your name or profile icon on the top right and select Account Settings > Business information > PayPal Merchant ID. To find the merchant ID of your sandbox account, follow the same instructions on sandbox.paypal.com. |
partnerClientId |
Your client ID. |
returnToPartnerUrl |
The URL you want to redirect your seller to after completing signup. Not specifying a return URL in this signup link follows the same behavior as not specifying a return URL when calling the Partner Referrals API. |
partnerLogoUrl |
The URL for the logo to be displayed during the onboarding process. This applies only if you're not loading the signup flow in a mini-browser. If you do not specify a logo URL in this signup link, the logo URL set on your account will be used. You can work with your account manager to set the logo URL on your account. |
merchantId |
The unique ID of the seller in your system. |
productIntentId |
Set to addipmt . |
features |
The permissions a seller grants you to operate on their PayPal account. If you do not specify features in this signup link, the features enabled for your account are used. Work with your account manager to enable features. |
showPermissions |
A Boolean that if set to true shows permissions in the onboarding flow. |
integrationType |
The type of integration between you and your sellers. To specify OAUTH_THIRD_PARTY , set to TO . |
Next steps
Add more payment methods