Integrate PayPal Checkout
Last updated: Jul 5th, 3:40am
How it works
Integrate standard Checkout to show the PayPal payment buttons. To extend your integration, see Customize your buyers' experience.
After you integrate standard Checkout, you can also offer the following options with some additional configuration:
- Pay Later
- Venmo
- PayPal Credit
This integration guide follows the code in this GitHub sample.
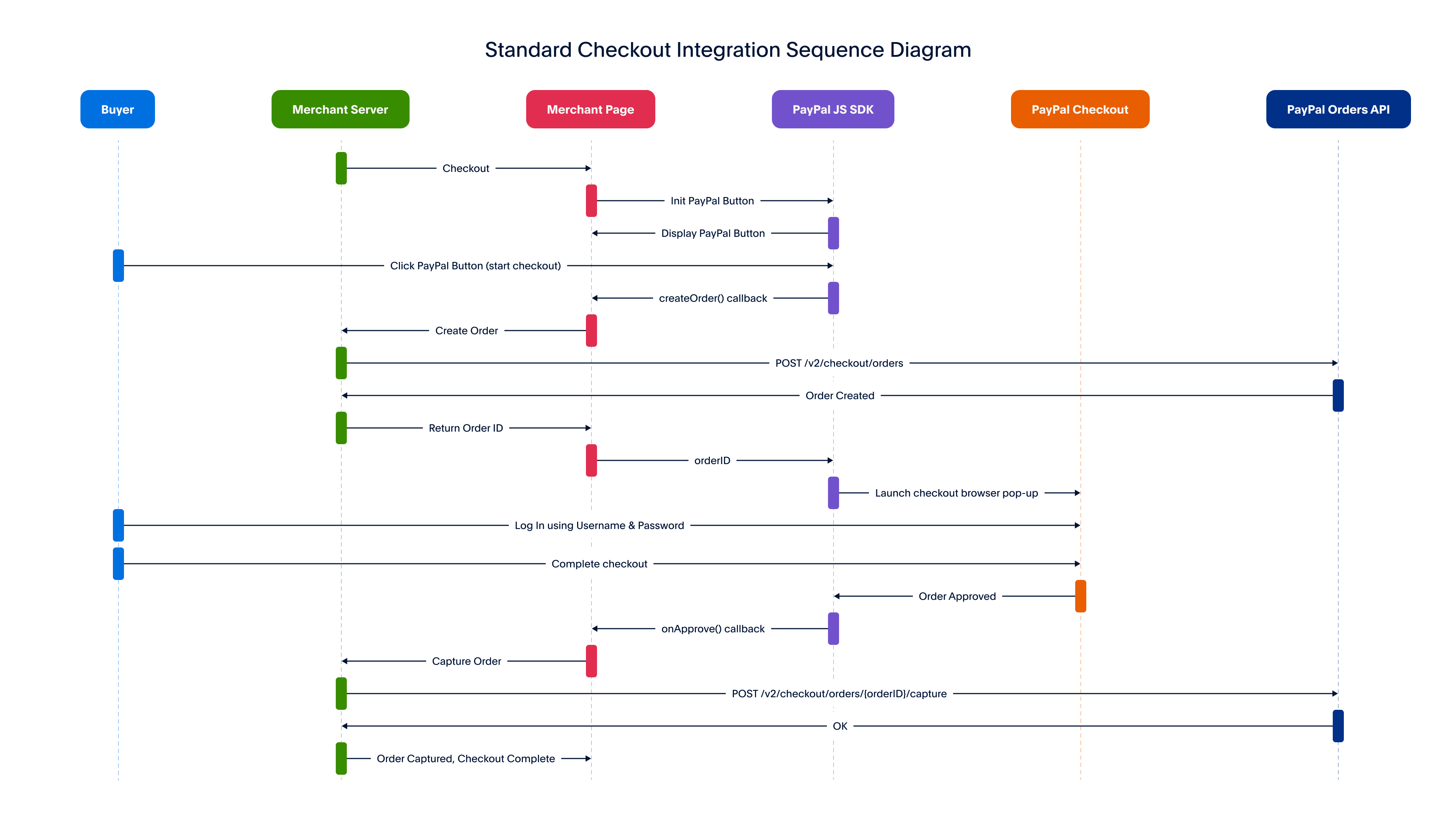
Know before you code
RequiredYou need the following to use this integration:
- Be an approved partner.
- Onboard your sellers before you begin the integration.
- Have an access token.
- Inform your sellers of PayPal's Seller Protection policy, so they are aware of use cases that invalidate that protection, such as shipping to an address other than the one in the transaction confirmation.
RequiredYou need a developer account to get sandbox credentials
PayPal uses the following REST API credentials, which you can get from the developer dashboard:
- Client ID: Authenticates your account with PayPal and identifies an app in your sandbox.
- Client secret: Authorizes an app in your sandbox. Keep this secret safe and don't share it.
RequiredYou'll need both PayPal and third-party tools
- JavaScript SDK: Adds PayPal-supported payment methods.
- Orders REST API: Create, update, retrieve, authorize, and capture orders.
- npm: Registry used to install third-party libraries.
OptionalExplore PayPal APIs with Postman
You can use Postman to explore and test PayPal APIs. Learn more in our Postman guide.
Set up your environment
Complete the following steps to set up your development environment.
1. Set up npm
This sample integration uses Node.js. You'll need to install npm to run the sample application. For more info, visit npm's documentation.
2. Install third-party libraries
You'll need to install the following third-party libraries to set up your integration. This sample command installs all libraries at the same time:
npm install dotenv express node-fetch
Third-party libraries | Description |
---|---|
dotenv | Separates your configuration and code by loading environment variables from a .env file into process.env. |
express | This lean Node.js web application framework supports web and mobile applications. |
node-fetch |
This function helps you make API requests, similar to window.fetch .
|
3. Verify Package.json
A package.json
file lists the packages and version numbers your app needs. You can share your package.json
file with other developers so they can use the same settings as you.
The following code sample shows a package.json
file for a PayPal integration. Compare this sample to the paste
in your project:
1{2 "name": "paypal-standard-integration",3 "description": "Sample Node.js web app to integrate PayPal Standard Checkout for online payments",4 "version": "1.0.0",5 "main": "server/server.js",6 "type": "module",7 "scripts":8 {9 "test": "echo \"Error: no test specified\" && exit 1",10 "start": "nodemon server/server.js",11 "format": "npx prettier --write **/*.{js,md}",12 "format:check": "npx prettier --check **/*.{js,md}",13 "lint": "npx eslint server/*.js --env=node && npx eslint client/*.js --env=browser"14 },15 "license": "Apache-2.0",16 "dependencies":17 {18 "dotenv": "^16.3.1",19 "express": "^4.18.2",20 "node-fetch": "^3.3.2"21 },22 "devDependencies":23 {24 "nodemon": "^3.0.1"25 }26}
Replace YOUR-SERVER-NAME.js
in main
with the name of your server file on lines 5 and 9.
If you're having trouble with your app, reinstall your local library and package files using npm install
.
If you're getting the following node error, include "type": "module"
in your package.json
file. This line isn't automatically added when package.json
is created.
Warning: To load an ES module, set "type": "module" in the package.json or use the .mjs extension (Use `node --trace-warnings ...` to show where the warning was created)
See line 6 of the sample package.json
file for an example.
4. Set up .env
A .env
file is a line-delimited text file that sets your local working environment variables. Use this .env
file to securely pass the client ID and client secret for your app.
The following code shows an example .env
file. Replace the PAYPAL-CLIENT-ID
and PAYPAL-CLIENT-SECRET
with values from your app:
1PAYPAL_CLIENT_ID=YOUR_CLIENT_ID_GOES_HERE2PAYPAL_CLIENT_SECRET=YOUR_SECRET_GOES_HERE
Note: View your client ID and client secret in the PayPal Developer Dashboard under Apps & Credentials.
Integrate back end
This section explains how to set up your back end to integrate standard Checkout.
Back-end process
- Your app creates an order on the back end by making a call to the Create Orders API endpoint.
- Your app moves the money when the payer confirms the order by making a call to to the Capture Payment for Order API endpoint on the back end.
- You can also customize the way you capture money. For example, you can use Authorize and Capture which places a hold on buyer’s money until the buyer is authorized. See the pages under Customize for more information.
Back-end code
This sample shows how to generate a PayPal-Auth-Assertion
header, add it to a node.js
file, and set up a /server/server.js
file to integrate with standard payments.
1. Generate a PayPal-Auth-Assertion header
Pass the PayPal-Auth-Assertion
header with standard Content-Type
, Authorization
, and PayPal-Request-ID
headers. Copy and modify the following code to generate the PayPal-Auth-Assertion
header.
1const clientId = "CLIENT-ID";2const sellerPayerId = "SELLER-PAYER-ID"3const jwt = getAuthAssertionValue(clientId, sellerPayerId);4console.log(jwt);56function getAuthAssertionValue(clientId, sellerPayerId) {7 const header = {8 "alg": "none"9 };10 const encodedHeader = base64url(header);11 const payload = {12 "iss": clientId,13 "payer_id": sellerPayerId14 };15 const encodedPayload = base64url(payload);16 return `${encodedHeader}.${encodedPayload}.`;17}1819function base64url(json) {20 return btoa(JSON.stringify(json))21 .replace(/=+$/, '')22 .replace(/\+/g, '-')23 .replace(/\//g, '_');24}
The preceding example contains period characters ( .
), which are required.
Modify the code:
- Replace
CLIENT-ID
with the client ID of the platform or marketplace from the PayPal developer dashboard. -
Replace
SELLER-PAYER-ID
with the payer ID or the email of the receiving seller's PayPal account.
Example functions to generate the PayPal-Auth-Assertion
header:
1const clientID = "Acuy17p2LcOf9RMv8SUVBb3wic3FPEP2NHFFqfSCBRFrNFdmbC1JQ0w8HIKRxW3RDy2R8QTL93eptFYl";2const merchantIDOrEmail = "identity_seller@paypal.com";3const auth1 = Buffer.from('{"alg":"none"}').toString("base64");4const auth2 = Buffer.from(`{"iss":${clientID},"payer_id":${merchantIDOrEmail}}`).toString("base64");5const authAssertionHeader = `${auth1}.${auth2}.`;
2. Set up your back end
The following code sample uses the server.js
file to set up the back-end to integrate with standard payments.
1import express from "express";2import fetch from "node-fetch";3import "dotenv/config";4import path from "path";56const7{8 PAYPAL_CLIENT_ID,9 PAYPAL_CLIENT_SECRET,10 PORT = 888811} = process.env;12const base = "https://api-m.sandbox.paypal.com";13const app = express();1415// host static files16app.use(express.static("client"));1718// parse post params sent in body in json format19app.use(express.json());2021/**22 * Generate an OAuth 2.0 access token for authenticating with PayPal REST APIs.23 * @see https://developer.paypal.com/api/rest/authentication/24 */25const generateAccessToken = async () =>26{27 try28 {29 if (!PAYPAL_CLIENT_ID || !PAYPAL_CLIENT_SECRET)30 {31 throw new Error("MISSING_API_CREDENTIALS");32 }33 const auth = Buffer.from(34 PAYPAL_CLIENT_ID + ":" + PAYPAL_CLIENT_SECRET,35 ).toString("base64");36 const response = await fetch(`${base}/v1/oauth2/token`,37 {38 method: "POST",39 body: "grant_type=client_credentials",40 headers:41 {42 Authorization: `Basic ${auth}`,43 },44 });4546 const data = await response.json();47 return data.access_token;48 }49 catch (error)50 {51 console.error("Failed to generate Access Token:", error);52 }53};5455/**56 * Create an order to start the transaction.57 * @see https://developer.paypal.com/docs/api/orders/v2/#orders_create58 */59const createOrder = async (cart) =>60{61 // use the cart information passed from the front-end to calculate the purchase unit details62 console.log(63 "shopping cart information passed from the frontend createOrder() callback:",64 cart,65 );6667 const accessToken = await generateAccessToken();68 const url = `${base}/v2/checkout/orders`;69 const payload = {70 intent: "CAPTURE",71 purchase_units: [72 {73 amount:74 {75 currency_code: "USD",76 value: "100.00",77 },78 }, ],79 };8081 const response = await fetch(url,82 {83 headers:84 {85 "Content-Type": "application/json",86 Authorization: `Bearer ${accessToken}`,87 // Uncomment one of these to force an error for negative testing (in sandbox mode only). Documentation:88 // https://developer.paypal.com/tools/sandbox/negative-testing/request-headers/89 // "PayPal-Mock-Response": '{"mock_application_codes": "MISSING_REQUIRED_PARAMETER"}'90 // "PayPal-Mock-Response": '{"mock_application_codes": "PERMISSION_DENIED"}'91 // "PayPal-Mock-Response": '{"mock_application_codes": "INTERNAL_SERVER_ERROR"}'92 },93 method: "POST",94 body: JSON.stringify(payload),95 });9697 return handleResponse(response);98};99100/**101 * Capture payment for the created order to complete the transaction.102 * @see https://developer.paypal.com/docs/api/orders/v2/#orders_capture103 */104const captureOrder = async (orderID) =>105{106 const accessToken = await generateAccessToken();107 const url = `${base}/v2/checkout/orders/${orderID}/capture`;108109 const response = await fetch(url,110 {111 method: "POST",112 headers:113 {114 "Content-Type": "application/json",115 Authorization: `Bearer ${accessToken}`,116 // Uncomment one of these to force an error for negative testing (in sandbox mode only). Documentation:117 // https://developer.paypal.com/tools/sandbox/negative-testing/request-headers/118 // "PayPal-Mock-Response": '{"mock_application_codes": "INSTRUMENT_DECLINED"}'119 // "PayPal-Mock-Response": '{"mock_application_codes": "TRANSACTION_REFUSED"}'120 // "PayPal-Mock-Response": '{"mock_application_codes": "INTERNAL_SERVER_ERROR"}'121 },122 });123124 return handleResponse(response);125};126127async function handleResponse(response)128{129 try130 {131 const jsonResponse = await response.json();132 return {133 jsonResponse,134 httpStatusCode: response.status,135 };136 }137 catch (err)138 {139 const errorMessage = await response.text();140 throw new Error(errorMessage);141 }142}143144app.post("/api/orders", async (req, res) =>145{146 try147 {148 // use the cart information passed from the front-end to calculate the order amount detals149 const150 {151 cart152 } = req.body;153 const154 {155 jsonResponse,156 httpStatusCode157 } = await createOrder(cart);158 res.status(httpStatusCode).json(jsonResponse);159 }160 catch (error)161 {162 console.error("Failed to create order:", error);163 res.status(500).json(164 {165 error: "Failed to create order."166 });167 }168});169170app.post("/api/orders/:orderID/capture", async (req, res) =>171{172 try173 {174 const175 {176 orderID177 } = req.params;178 const179 {180 jsonResponse,181 httpStatusCode182 } = await captureOrder(orderID);183 res.status(httpStatusCode).json(jsonResponse);184 }185 catch (error)186 {187 console.error("Failed to create order:", error);188 res.status(500).json(189 {190 error: "Failed to capture order."191 });192 }193});194195// serve index.html196app.get("/", (req, res) =>197{198 res.sendFile(path.resolve("./client/checkout.html"));199});200201app.listen(PORT, () =>202{203 console.log(`Node server listening at http://localhost:${PORT}/`);204});
Understand the server.js code sample
The following section explains key parts of the server.js
code sample.
In the back-end code, you’ll need to modify as follows:
- Change
BN-CODE
to your PayPal Partner Attribution ID. - Change
ACCESS-TOKEN
to your access token. - Change
PAYPAL-AUTH-ASSERTION
to thePaypal-auth-assertion
header you generated in the Generate a PayPal-Auth-Assertion header section.
Declare imports
This section of code imports the dotenv dependency, the express module, the node fetch module and the path module.
1import express from "express";2import fetch from "node-fetch";3import "dotenv/config";4import path from "path";
Set up port and server
This section of code sets up the port to run your server and starts the express Node.js web application framework. It also retreives variables and sets the base sandbox URL.
1const { PAYPAL_CLIENT_ID, PAYPAL_CLIENT_SECRET, PORT = 8888 } = process.env;2const base = "https://api-m.sandbox.paypal.com";3const app = express();
- Line 1 uses the DotEnv library to import and declare the client ID and client secret from your .env file.
- Line 2 declares the base URL for PayPal's sandbox API.
Additional functions
This section of the code defines a file directory for static files and calls the express.json function to parse JSON response bodies.
1// host static files2app.use(express.static("client"));34// parse post params sent in body in json format5app.use(express.json());
Generate access token
You need an access token to authenticate all REST API requests. The following code sample makes a POST call to the /v1/oauth2/
token endpoint to create an access token.
1/**2 * Generate an OAuth 2.0 access token for authenticating with PayPal REST APIs.3 * @see https://developer.paypal.com/api/rest/authentication/4 */5const generateAccessToken = async () =>6{7 try8 {9 if (!PAYPAL_CLIENT_ID || !PAYPAL_CLIENT_SECRET)10 {11 throw new Error("MISSING_API_CREDENTIALS");12 }13 const auth = Buffer.from(14 PAYPAL_CLIENT_ID + ":" + PAYPAL_CLIENT_SECRET,15 ).toString("base64");16 const response = await fetch(`${base}/v1/oauth2/token`,17 {18 method: "POST",19 body: "grant_type=client_credentials",20 headers:21 {22 Authorization: `Basic ${auth}`,23 },24 });2526 const data = await response.json();27 return data.access_token;28 }29 catch (error)30 {31 console.error("Failed to generate Access Token:", error);32 }33};
- Lines 10-12 combine the
PAYPAL_CLIENT_ID
andPAYPAL_CLIENT_SECRET
as a key-value pair. - Lines 13-18 define a response that makes a
POST
call to the/v1/oauth2/token
API endpoint to generate an access token. - Lines 21-22 establish a listener to capture the response data from the request and return the
access_token
.
Create order
Create an order to start a payment between a payer and a seller by making a POST
request to /v2/checkout/orders
.
If you process payments that require Strong Customer Authentication, you need to provide additional context with payment indicators.
1/**2 * Create an order to start the transaction.3 * @see https://developer.paypal.com/docs/api/orders/v2/#orders_create4 */5const createOrder = async (cart) =>6{7 // use the cart information passed from the front-end to calculate the purchase unit details8 console.log(9 "shopping cart information passed from the frontend createOrder() callback:",10 cart,11 );1213 const accessToken = await generateAccessToken();14 const url = `${base}/v2/checkout/orders`;15 const payload = {16 intent: "CAPTURE",17 purchase_units: [18 {19 amount:20 {21 currency_code: "USD",22 value: "100.00",23 },24 }, ],25 };2627 const response = await fetch(url,28 {29 headers:30 {31 "Content-Type": "application/json",32 Authorization: `Bearer ${accessToken}`,33 // Uncomment one of these to force an error for negative testing (in sandbox mode only). Documentation:34 // https://developer.paypal.com/tools/sandbox/negative-testing/request-headers/35 // "PayPal-Mock-Response": '{"mock_application_codes": "MISSING_REQUIRED_PARAMETER"}'36 // "PayPal-Mock-Response": '{"mock_application_codes": "PERMISSION_DENIED"}'37 // "PayPal-Mock-Response": '{"mock_application_codes": "INTERNAL_SERVER_ERROR"}'38 “PayPal - Partner - Attribution - Id”: “BN - CODE”,39 “PayPal - Auth - Assertion”: “PAYPAL - AUTH - ASSERTION”,40 },41 method: "POST",42 body: JSON.stringify(payload),43 });4445 return handleResponse(response);46};
- Line 5 calls the
createOrder
function and uses the cart information from the front-end to calculate the purchase units for the order. - Line 12 establishes a listener to capture the
accessToken
from thegenerateAccessToken()
function later in the API call. - Lines 13-38 create an order by sending a
POST
request to the Orders v2 API, using theaccessToken
.
See the Create order endpoint of the PayPal Orders v2 API for sample responses and other details.
Processor response codes
Payment processors return processor response codes when they receive a transaction request. For advanced card payments, the code displays in the authorization object under the response_code
field.
1"processor_response": {2 "avs_code": "Y",3 "cvv_code": "S",4 "payment_advice_code": "",5 "response_code": "0000"6}
If an external payment processor declines a transaction, PayPal returns a HTTP 201 CREATED
status code and a status of DECLINED
in the capture status.
See the Orders API response_code
object to get the processor response code for the non-PayPal payment processor errors.
Capture payment
Capture an order to move money from the payer to the merchant by making a POST
call to the /v2/checkout/orders/ORDER-ID/capture
endpoint.
1/**2 * Capture payment for the created order to complete the transaction.3 * @see https://developer.paypal.com/docs/api/orders/v2/#orders_capture4 */5const captureOrder = async (orderID) =>6 {7 const accessToken = await generateAccessToken();8 const url = `${base}/v2/checkout/orders/${orderID}/capture`;910 const response = await fetch(url,11 {12 method: "POST",13 headers:14 {15 "Content-Type": "application/json",16 Authorization: `Bearer ${accessToken}`,17 // Uncomment one of these to force an error for negative testing (in sandbox mode only). Documentation:18 // https://developer.paypal.com/tools/sandbox/negative-testing/request-headers/19 // "PayPal-Mock-Response": '{"mock_application_codes": "INSTRUMENT_DECLINED"}'20 // "PayPal-Mock-Response": '{"mock_application_codes": "TRANSACTION_REFUSED"}'21 // "PayPal-Mock-Response": '{"mock_application_codes": "INTERNAL_SERVER_ERROR"}'22 “PayPal - Partner - Attribution - Id”: “BN - CODE”,23 “PayPal - Auth - Assertion”: “PAYPAL - AUTH - ASSERTION”,24 },25 });
- Line 5 establishes a listener to capture the
accessToken
from thegenerateAccessToken()
function later in the API call. - Line 8 declares the URL of the Capture API endpoint using the Order ID generated from the Create Order endpoint.
- Lines 10-16 define a response that makes a
POST
call to the/v2/checkout/orders/ORDER-ID/capture
endpoint to capture the order, using theaccessToken
. - Lines 19-21 include mock responses for negative testing in the sandbox.
Sample capture response
This code sample shows a response to a POST
call to /v2/checkout/orders/ORDER-ID/capture
. The response is the orderData
retrieved in the create order section.
1{2 "id": "5O190127TN364715T",3 "status": "COMPLETED",4 "payment_source":5 {6 "paypal":7 {8 "name":9 {10 "given_name": "Firstname",11 "surname": "Lastname"12 },13 "email_address": "payer@example.com",14 "account_id": "QYR5Z8XDVJNXQ",15 },16 },17 "purchase_units": [18 {19 "reference_id": "d9f80740-38f0-11e8-b467-0ed5f89f718b",20 "shipping":21 {22 "address":23 {24 "address_line_1": "123 Main St.",25 "admin_area_2": "Anytown",26 "admin_area_1": "CA",27 "postal_code": "12345",28 "country_code": "US",29 },30 },31 "payments":32 {33 "captures": [34 {35 "id": "3C679366HH908993F",36 "status": "COMPLETED",37 "amount":38 {39 "currency_code": "USD",40 "value": "100.00",41 },42 "seller_protection":43 {44 "status": "ELIGIBLE",45 "dispute_categories": [46 "ITEM_NOT_RECEIVED",47 "UNAUTHORIZED_TRANSACTION",48 ],49 },50 "final_capture": true,51 "disbursement_mode": "INSTANT",52 "seller_receivable_breakdown":53 {54 "gross_amount":55 {56 "currency_code": "USD",57 "value": "100.00",58 },59 "paypal_fee":60 {61 "currency_code": "USD",62 "value": "3.00",63 },64 "net_amount":65 {66 "currency_code": "USD",67 "value": "97.00",68 },69 },70 "create_time": "2018-04-01T21:20:49Z",71 "update_time": "2018-04-01T21:20:49Z",72 "links": [73 {74 "href": "https://api-m.paypal.com/v2/payments/captures/3C679366HH908993F",75 "rel": "self",76 "method": "GET",77 },78 {79 "href": "https://api-m.paypal.com/v2/payments/captures/3C679366HH908993F/refund",80 "rel": "refund",81 "method": "POST",82 }, ],83 }, ],84 },85 }, ],86 "payer":87 {88 "name":89 {90 "given_name": "Firstname",91 "surname": "Lastname",92 },93 "email_address": "payer@example.com",94 "payer_id": "QYR5Z8XDVJNXQ",95 },96 "links": [97 {98 "href": "https://api-m.paypal.com/v2/checkout/orders/5O190127TN364715T",99 "rel": "self",100 "method": "GET",101 }, ],102}
- Line 2 shows the ID for this
orderData
object. - Lines 4-16 pass details about the payment source.
- Lines 17-85 pass the
purchase_units
in this transaction. Each purchase unit represents either a full or partial order and establishes a contract between a payer and a merchant. - Line 19 passes the
reference_id
that identifies the purchase unit in this payment response. - Lines 22-29 pass details about the shipping address.
- Line 31 declares the payments object that passes the payment details for this capture request.
- Line 37 declares the captures object that passes details about the captured payments for this request.
- Lines 33-83 pass the payment capture details, such as the capture identifier
id
,amount
,disbursement_mode
, andnet_amount
. - Lines 72-82 pass the HATEOAS details of the capture response. See the REST API Response Reference for more details about HATEOAS.
- Lines 86-95 pass details about the payer.
- Lines 96-101 pass the HATEOAS details for the orders response.
Handle responses
The handleResponse
function sets up a listener for API responses.
1async function handleResponse(response) {2 try {3 const jsonResponse = await response.json();4 return {5 jsonResponse,6 httpStatusCode: response.status,7 };8 } catch (err) {9 const errorMessage = await response.text();10 throw new Error(errorMessage);11 }12}1314app.post("/api/orders", async (req, res) => {15 try {16 // use the cart information passed from the front-end to calculate the order amount detals17 const { cart } = req.body;18 const { jsonResponse, httpStatusCode } = await createOrder(cart);19 res.status(httpStatusCode).json(jsonResponse);20 } catch (error) {21 console.error("Failed to create order:", error);22 res.status(500).json({ error: "Failed to create order." });23 }24});2526app.post("/api/orders/:orderID/capture", async (req, res) => {27 try {28 const { orderID } = req.params;29 const { jsonResponse, httpStatusCode } = await captureOrder(orderID);30 res.status(httpStatusCode).json(jsonResponse);31 } catch (error) {32 console.error("Failed to create order:", error);33 res.status(500).json({ error: "Failed to capture order." });34 }35});
- Line 1 creates a function which returns a HTTP status code response. Error status codes send an error message.
- Line 14 makes a
POST
call to theapi/orders/
endpoint and returns an HTTP status code response. Errors status codes send an error message. - Line 26 makes a
POST
call to theapi/orders/:orderID/capture
endpoint and returns an HTTP status code response for the particular order. Error status codes send an error message.
Integrate front end
Set up your front-end to integrate standard checkout payments.
Front-end process
- Your app displays the PayPal checkout buttons.
- Your app calls server endpoints to create the order and capture payment.
Front-end code
This example uses the checkout.html file to show how to set up the front end to integrate standard payments.
/client/checkout.html
handles the client-side logic and defines how the PayPal front-end components connect with the back end. Use this file to set up the PayPal checkout using the JavaScript SDK and handle the payer's interactions with the PayPal checkout button.
- /client/checkout.html
- /client/app.js
1<html lang="en">2 <head>3 <meta charset="UTF-8">4 <meta name="viewport" content="width=device-width, initial-scale=1.0">5 <title>PayPal JS SDK Standard Integration</title>6 </head>7 <body>8 <div id="paypal-button-container"></div>9 <p id="result-message"></p>10 <!-- Replace the "test" client-id value with your client-id -->11 <script src="https://www.paypal.com/sdk/js?client-id={CLIENT_ID}&merchant-id={SELLER_PAYER_ID}&components=buttons" data-partner-attribution-id="{PARTNER_BN_CODE}"></script>12 <script src="app.js"></script>13 </body>14</html>
checkout.html
This section explains the checkout.html
code sample.
PayPal Buttons JavaScript
This code sample calls the PayPal JavaScript SDK
1<!DOCTYPE html>2<html lang="en">3 <head>4 <meta charset="UTF-8">5 <meta name="viewport" content="width=device-width, initial-scale=1.0">6 <title>PayPal JS SDK Standard Integration</title>7 </head>8 <body>9 <div id="paypal-button-container"></div>10 <p id="result-message"></p>11 <!-- Replace the "test" client-id value with your client-id -->12 <script src="https://www.paypal.com/sdk/js?client-id={CLIENT_ID}&merchant-id={SELLER_PAYER_ID}&components=buttons" data-partner-attribution-id="{PARTNER_BN_CODE}"></script>13 <script src="app.js"></script>14 </body>15</html>
- Line 9 displays the PayPal buttons.
- Line 10 displays the transaction results.
- Line 12 calls the PayPal SDK.
- line 13 runs the app.js script to start a checkout transaction.
app.js
This section explains the app.js
code sample.
PayPal Buttons JavaScript
This code sample calls the JavaScript SDK that defines the PayPal buttons.
1window.paypal.Buttons({{…}}).render(“#paypal-button-container”);
Create order for PayPal Button
This code sample defines the createOrder()
function.
1async createOrder() {2 try {3 const response = await fetch("/api/orders", {4 method: "POST",5 headers: {6 "Content-Type": "application/json",7 "Authorization”: “Bearer ACCESS-TOKEN",8 “PayPal-Partner-Attribution-Id”: “BN-CODE”9 “PayPal-Auth-Assertion”: “PAYPAL-AUTH-ASSERTION”10 },11 // use the "body" param to optionally pass additional order information12 // like product ids and quantities13 body: JSON.stringify({14 cart: [15 {16 id: "YOUR_PRODUCT_ID",17 quantity: "YOUR_PRODUCT_QUANTITY",18 },19 ],20 }),21 });2223 const orderData = await response.json();2425 if (orderData.id) {26 return orderData.id;27 } else {28 const errorDetail = orderData?.details?.[0];29 const errorMessage = errorDetail30 ? `${errorDetail.issue} ${errorDetail.description} (${orderData.debug_id})`31 : JSON.stringify(orderData);3233 throw new Error(errorMessage);34 }35 } catch (error) {36 console.error(error);37 resultMessage(`Could not initiate PayPal Checkout...<br><br>${error}`);38 }39 },
- Line 3 creates the order by calling the
api/orders
endpoint - Lines 14-21 pass the SKU and quantity for the product in the cart. See the
createOrder
section of the JavaScript SDK reference guide for more information about creating orders. See the Orders REST API documentation for additional customization options.
Capture payment when approved
This code sample defines a POST
call to /api/orders/orderID/capture
. The orderID is passed from the SDK when you run the onCreate()
call.
1async onApprove(data, actions) {2 try {3 const response = await fetch(/api/orders / $ {4 data.orderID5 }6 /capture, {7 method: “POST”,8 headers: {9 “Content - Type”: “application / json”,10 "Authorization”: “Bearer ACCESS-TOKEN",11 “PayPal - Partner - Attribution - Id”: “BN - CODE”“ PayPal - Auth - Assertion”: “PAYPAL - AUTH - ASSERTION”12 },13 }14 );15 const orderData = await response.json();16 // Three cases to handle:17 // (1) Recoverable INSTRUMENT_DECLINED -> call actions.restart()18 // (2) Other non-recoverable errors -> Show a failure message19 // (3) Successful transaction -> Show confirmation or thank you message2021 const errorDetail = orderData?.details?.[0];2223 if (errorDetail?.issue === "INSTRUMENT_DECLINED") {24 // (1) Recoverable INSTRUMENT_DECLINED -> call actions.restart()25 // recoverable state, per https://developer.paypal.com/docs/checkout/standard/customize/handle-funding-failures/26 return actions.restart();27 }28 else if (errorDetail) {29 // (2) Other non-recoverable errors -> Show a failure message30 throw new Error(`${errorDetail.description} (${orderData.debug_id})`);31 }32 else if (!orderData.purchase_units) {33 throw new Error(JSON.stringify(orderData));34 }35 else {36 // (3) Successful transaction -> Show confirmation or thank you message37 // Or go to another URL: actions.redirect('thank_you.html');38 const transaction =39 orderData?.purchase_units?.[0]?.payments?.captures?.[0] ||40 orderData?.purchase_units?.[0]?.payments?.authorizations?.[0];41 resultMessage(42 `Transaction ${transaction.status}: ${transaction.id}<br><br>See console for all available details`,43 );44 console.log(45 "Capture result",46 orderData,47 JSON.stringify(orderData, null, 2),48 );49 }50}51catch (error) {52 console.error(error);53 resultMessage(54 `Sorry, your transaction could not be processed...<br><br>${error}`,55 );56}57},58})
- Line 3 creates the order by calling the
api/orders/capture
endpoint - Line 11 sets up a listener for responses from the
api/orders/capture
call. - Lines 17-43 parse the order details from the response.
- Line 31 calls a response function to display the transaction results.
Display transaction results
This code sample defines an example resultMessage()
function.
1// Example function to show a result to the user. Your site’s UI library can be used instead.function resultMessage(message) {const container = document.querySelector(“#result-message”);container.innerHTML = message;
Render the PayPal buttons
This code sample renders the PayPal buttons.
1.render("#paypal-button-container");
Run your app
- Run
npm start
to run your server again. - Open your browser and navigate to
localhost:8888
- When your server is running, proceed to the next section to test your integration.
Design guidance
- Show the PayPal button on all pages that start the checkout process.
- Give PayPal equal prominence and function for your payers alongside all other acceptance marks, including cards, split tender, buy online, and pickup in-store.
- It should take no more than 2 steps for your payer to pay and complete their order when they return to your site.
- Leave room on your checkout page for the Debit or Credit Card button to expand. If you place the payment buttons too low on the page, payers won't be able to access the drop-down credit card form fields.
- If the Debit or Credit Card drop-down form isn't available when your payers check out, the PayPal guest checkout shows up in a pop-up window.
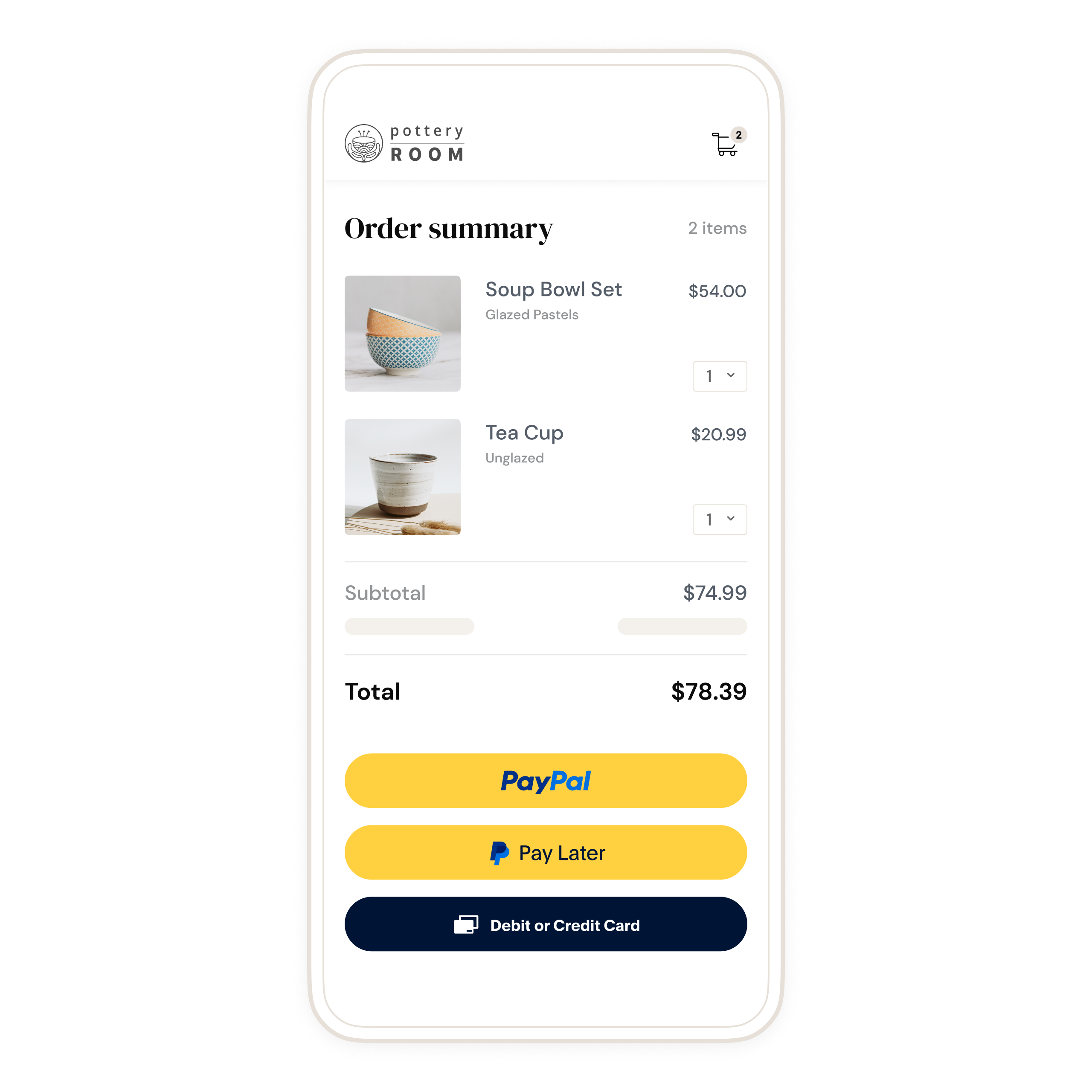
Test integration
Before going live, test your integration in the sandbox environment.
Learn more about the following resources on card testing:
- Successful payments by using test card numbers.
- Card error scenarios by using rejection triggers.
Use the credit card generator to generate test credit cards for sandbox testing.
Test the following use cases before going live:
Test a purchase as a payer
- Select the PayPal button on your checkout page.
- Log in using one of your personal sandbox accounts. This ensures the payments will be sent to the correct account. Make sure that you use the sandbox business account that corresponds to the REST app you are using.
- Note the purchase amount in the PayPal checkout window.
- Approve the purchase with the Pay Now button. The PayPal window closes and redirects you to your page, indicating that the transaction was completed.
Confirm the money reached the business account
- Log in to the PayPal sandbox using the sandbox business account that received the payment. Remember that the SDK source now uses a sandbox client ID from one of your own REST apps, and not the default test ID.
- In Recent Activity, confirm that the sandbox business account received the money, subtracting any fees.
- Log out of the account.
Test a purchase as a card payment
- Go to the checkout page for your integration.
- Generate a test card using the credit card generator.
- Enter the card details in the hosted field, including the name on the card, billing address, and 2-character country code. Then, submit the order.
- Confirm that the order was processed.
- Log in to your merchant sandbox account and navigate to the activity page to ensure the payment amount shows up in the account.
Go live
Follow this checklist to take your application live:
- Log into the PayPal Developer Dashboard with your PayPal business account.
- Obtain your live credentials.
- Include the new credentials in your integration and Update your PayPal endpoint.
See Move your app to production for more details.
Customize
Add more payment methods or customize your integration.